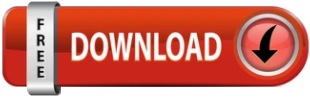
if turn <= prob_heads: if num_flips % 2 != 0: player_one_wins += 1 win += 1 Each time a turn is taken, we add one to our num_flips counter. Each “turn” constitutes a flip of the coin, which is a randomly selected decimal value between 0 and 1. While win remains equal to zero, the players continue to flip the coin. We also specify a win condition “win” that will help us know when a game has been completed. This both keeps track of how the long the game has gone on, and will help us to determine who the winner is once a heads has been flipped. Now, for every game in the specified number of simulations (e.g., range(0, num_games), we will count the number of coin flips starting from zero. for n in range(0,num_games): num_flips = 0 win = 0 while win = 0: turn = np.random.uniform(0,1) num_flips += 1 We also set a global counter for the number of games won by Player 1, to begin at 0 for each round of simulations. Obviously, we can enter custom probabilities here as we like. We’ll set a default probability of heads to 0.5, in case we simply want to specify a number of games using a fair coin to see what results. We define the name of our function, and specify our two arguments. We begin by importing numpy, as we can utilize its random choice functionality to simulate the coin-flipping mechanism for this game.

import numpy as np def P1_win_prob_weighted_coin_game(num_games, prob_heads = 0.5): player_one_wins = 0 Now, let’s walk through this briefly step-by-step. We can simulate this game with the following code: import numpy as np def P1_win_prob_weighted_coin_game(num_games, prob_heads=.5): player_one_wins = 0 for n in range(0,num_games): num_flips = 0 win = 0 while win = 0: turn = np.random.uniform(0,1) num_flips += 1 if turn <= prob_heads: if num_flips % 2 != 0: player_one_wins += 1 win += 1 return float(player_one_wins)/float(num_games) Let’s call this function “P1_win_prob_weighted_coin_game” Let’s write a function that takes in two arguments: 1.) the number of games to be played, and 2.) the probability that a coin flip will result in heads (set to a default of 0.5 or 50%). We can explore this problem with a simple function in python.
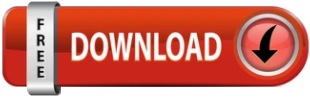